Lab Resources
Compound action workflow syntax
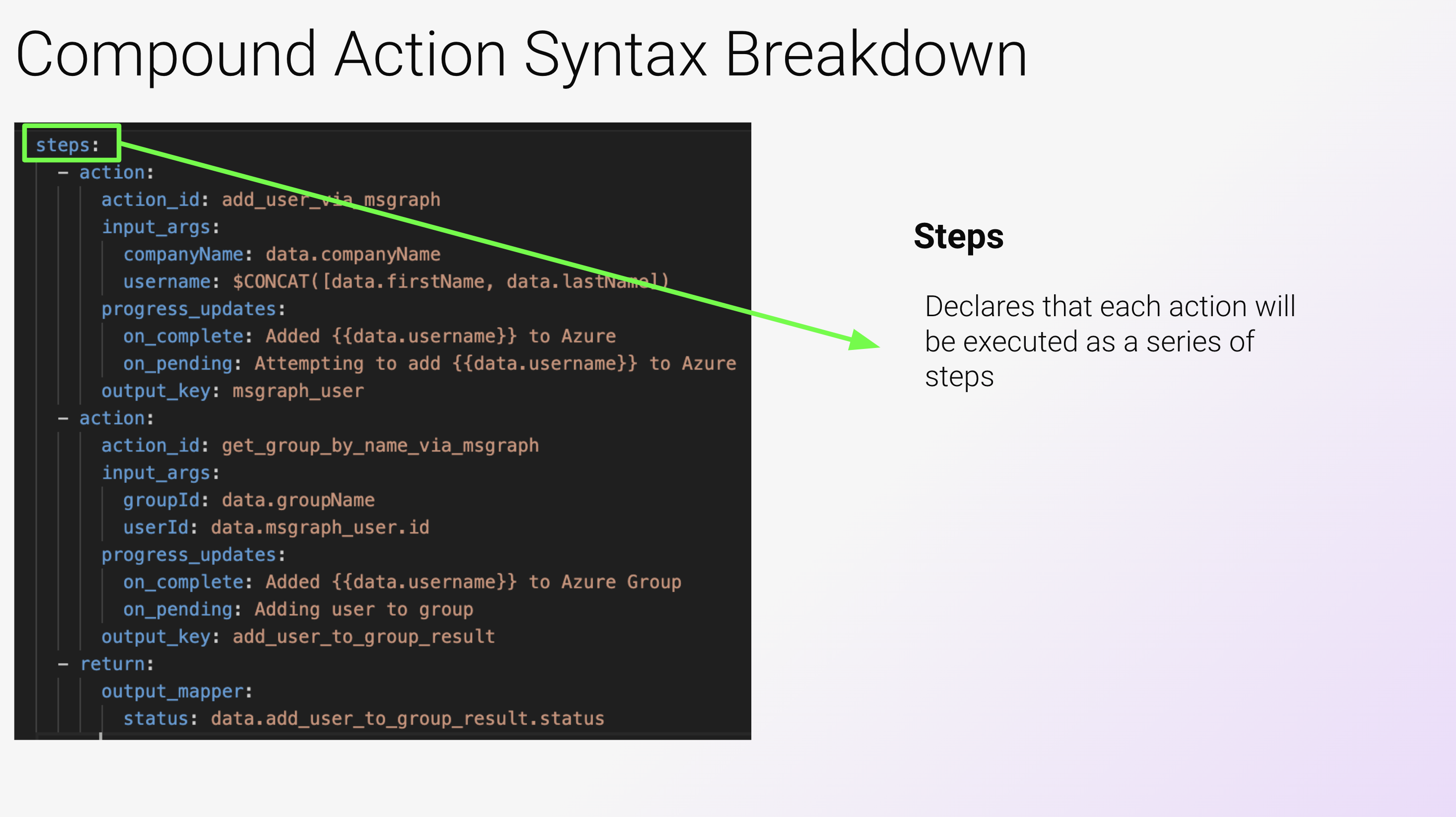
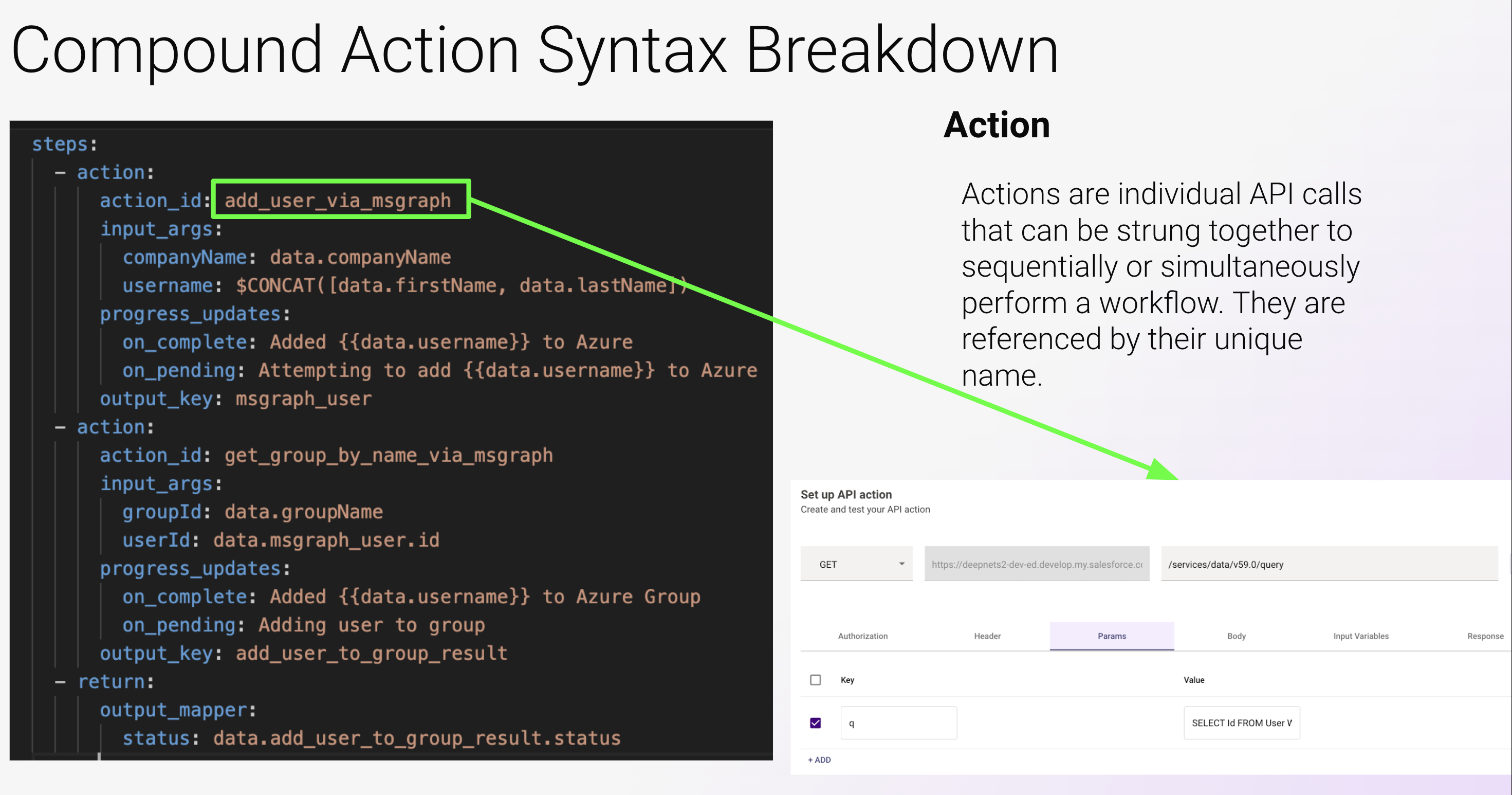
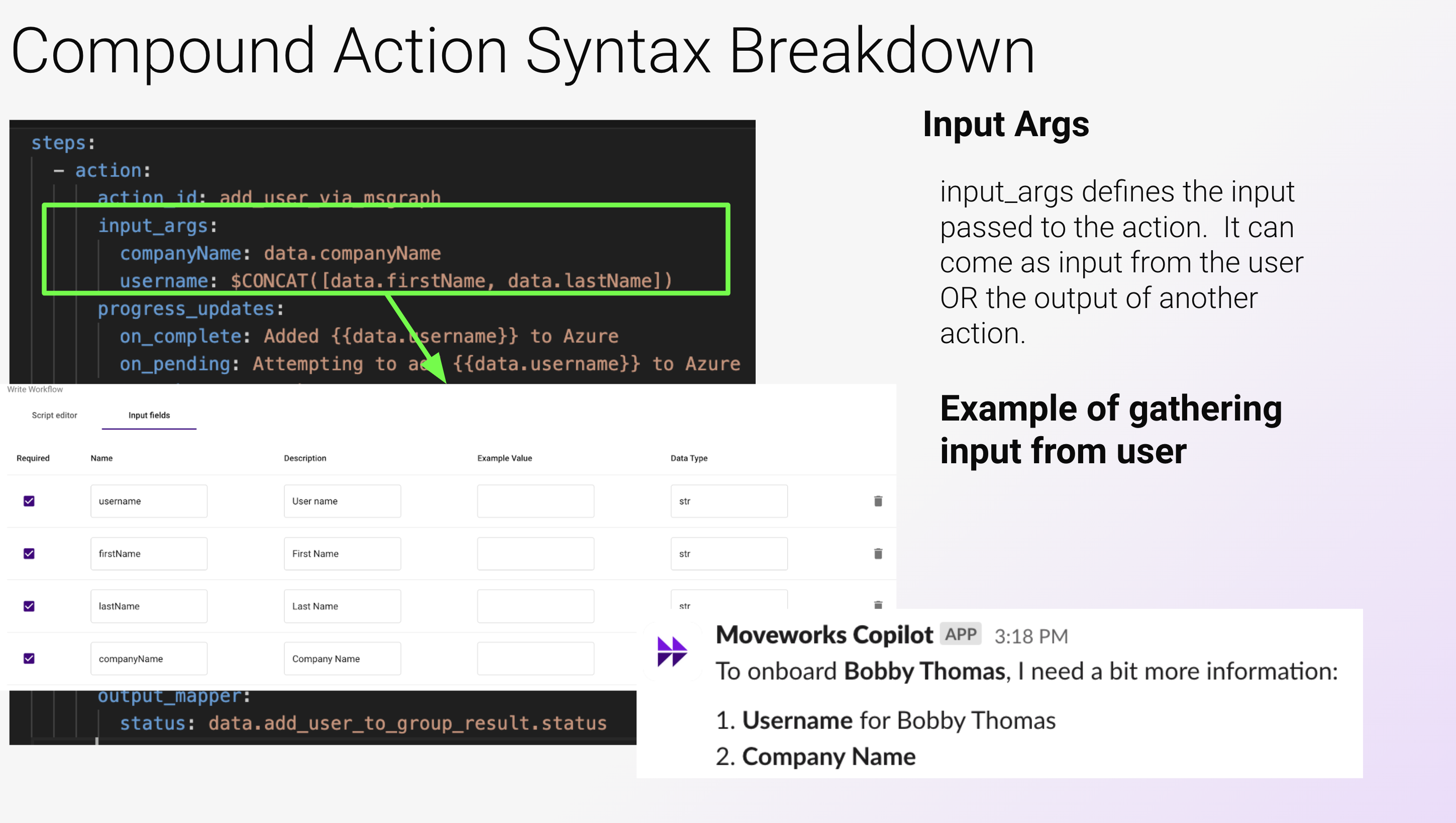
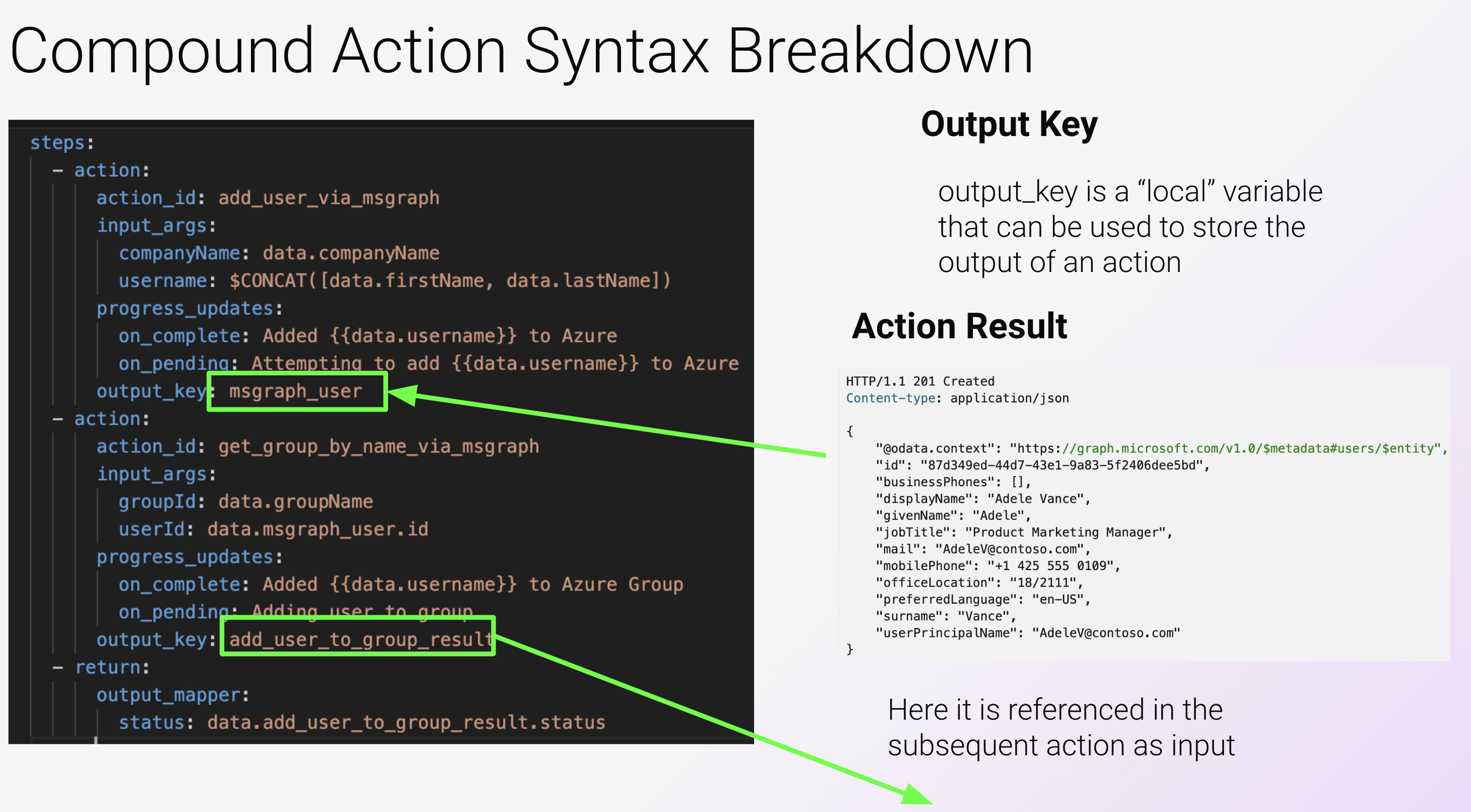
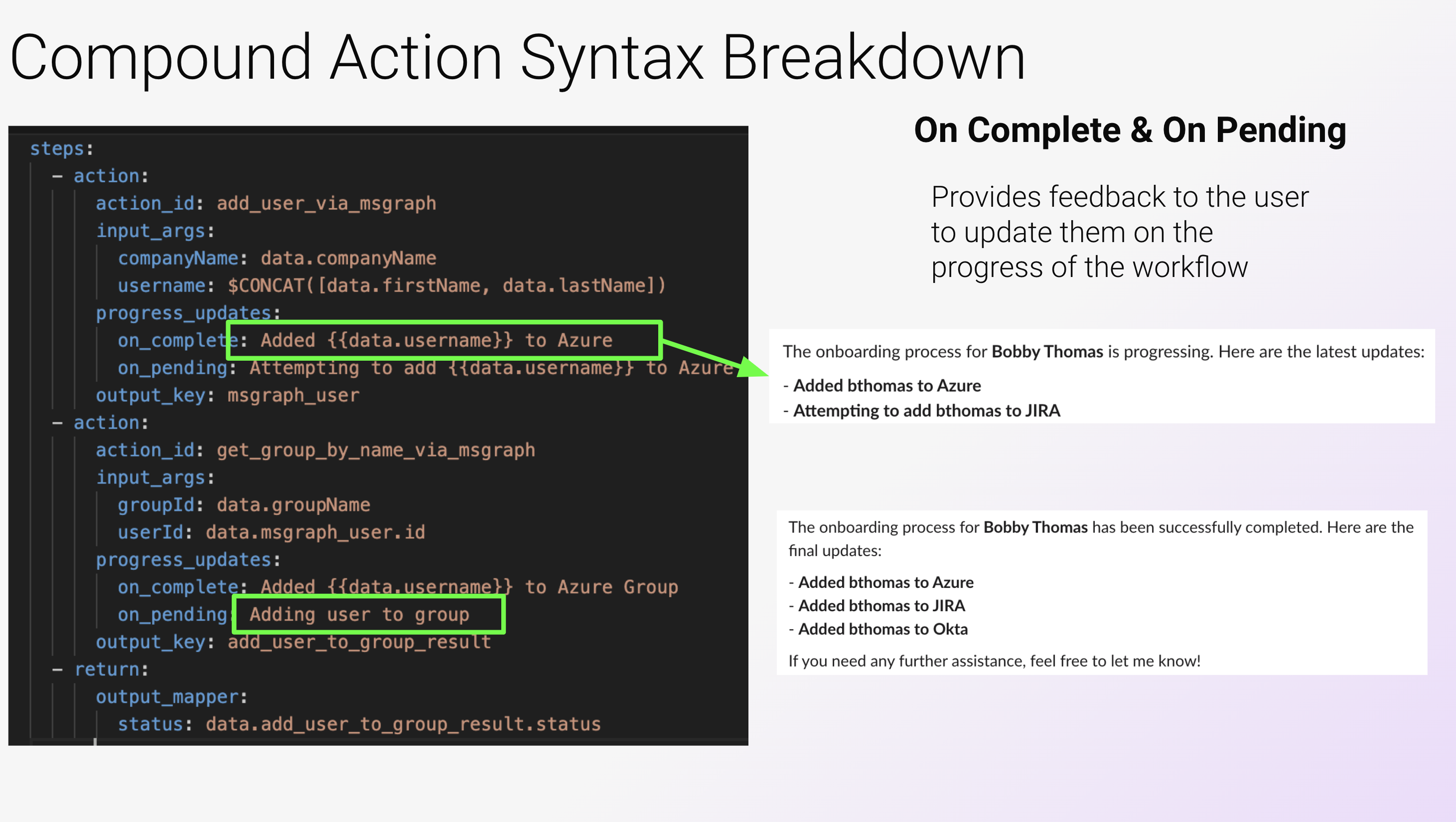
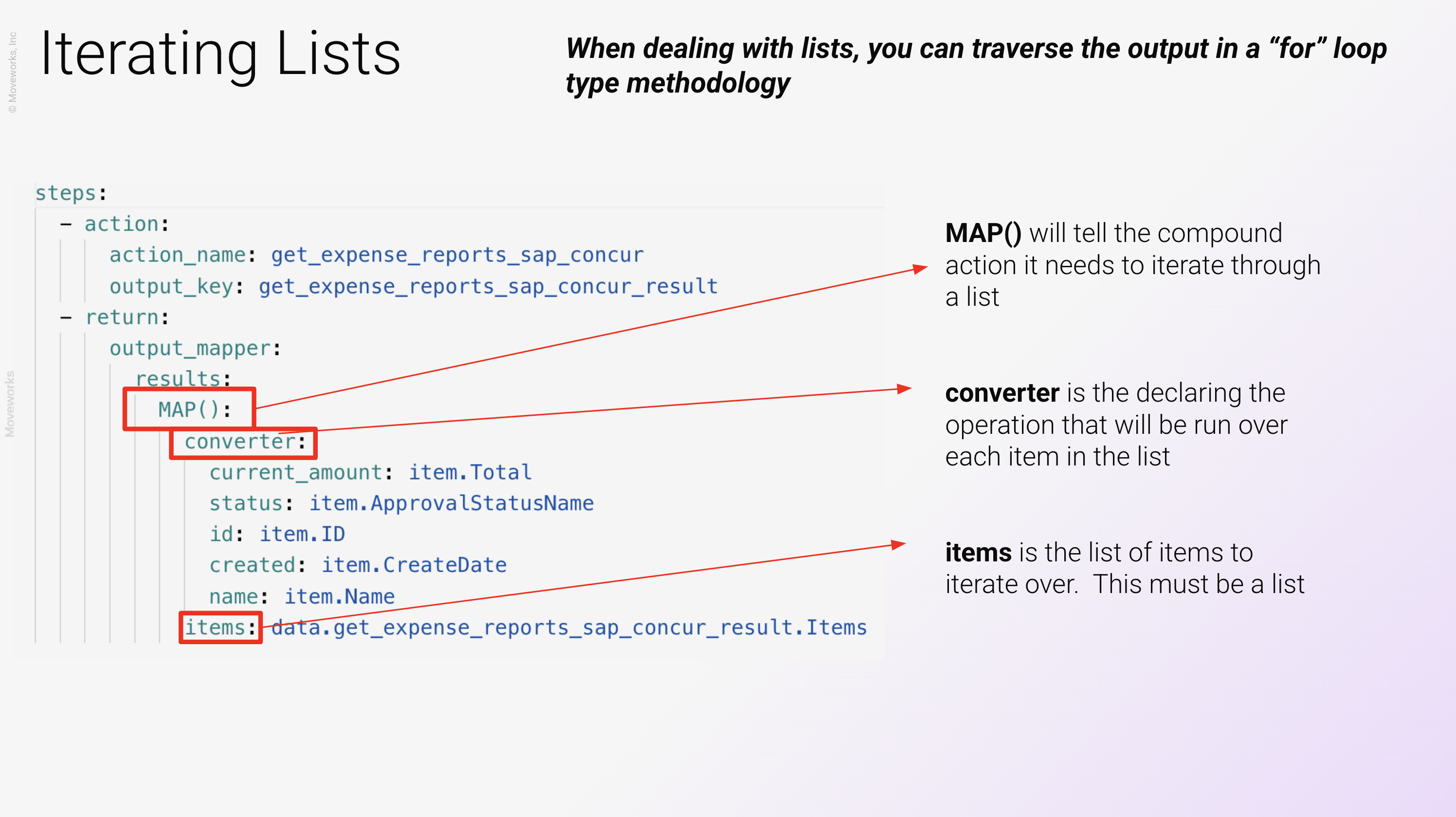
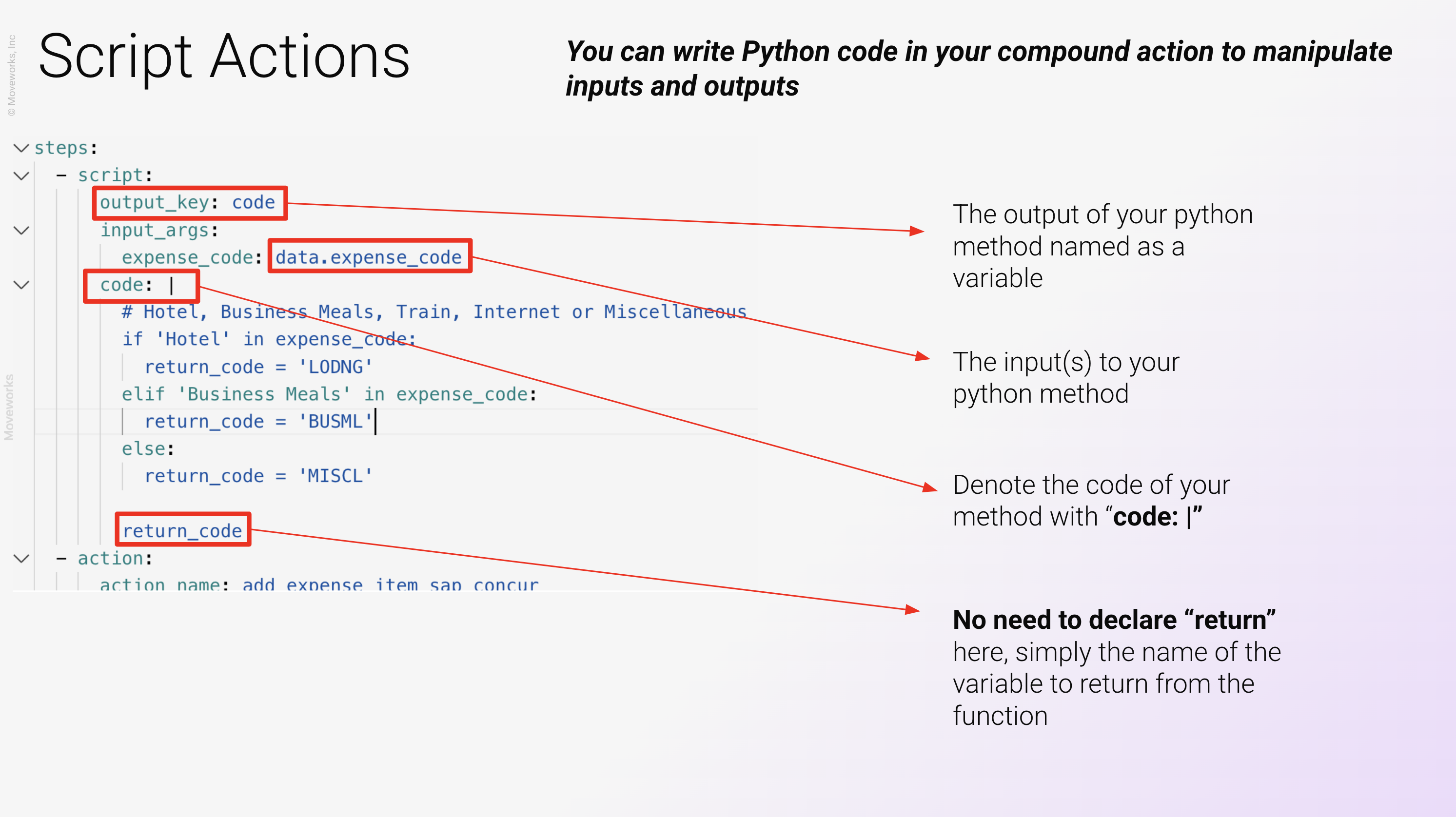
You can see more about API Script actions and APIThon here
Accessing data payloads in workflows
All data is accessible under the root field “data” as seen below in the JSON example.
Fields at the “data” level (description, subject, and type) can be accessed by referencing the next level using a “.”
Examples:
- To access description the syntax would be: data.description
- To access the jira issue key the syntax would be: data.jira_issue.key
API calls
Salesforce
Create case
curl --location 'https://deepnets2-dev-ed.develop.my.salesforce.com/services/data/v59.0/sobjects/Case' \
--header 'Content-Type: application/json' \
--data '{
"AccountId": "{{account_id}}",
"Subject": "Customer says its not working",
"Description": "testing it out again"
}'
Update case
curl --location --request PATCH 'https://deepnets2-dev-ed.develop.my.salesforce.com/services/data/v59.0/sobjects/Case/500aj00000Jl4reAAB'
--header 'Content-Type: application/json' \
--data '{"Comments":"https://deep-nets-team.atlassian.net/browse/KAN-11"}'
Jira
JIRA - Create issue
Hint: JIRA uses Basic Authentication. The username will be your JIRA username from your trial instance and the password will be the token you generated earlier in the exercise.
curl --location 'https://deep-nets-team.atlassian.net/rest/api/2/issue' \
--header 'Content-Type: application/json' \
--data '{
"fields":
{
"summary": "Add something to the Kanban board",
"description": "testing it out again",
"project":
{
"key": "KAN"
},
"issuetype":
{
"name": "Task"
}
},
"update": {}
}'
Azure
Get groups
curl --location 'https://graph.microsoft.com/v1.0/groups?%24top=10'
Filter groups
curl --location 'https://graph.microsoft.com/v1.0/groups?%24filter=startswith(displayName%2C%20%27demo%27')
Add user to group
curl --location 'https://graph.microsoft.com/v1.0/groups/{{group_id}}/members/$ref' \
--header 'Content-Type: application/json' \
--data-raw '{
"@odata.id": "https://graph.microsoft.com/v1.0/directoryObjects/{{user_id}}"
}'
Get groups for a user
curl --location 'https://graph.microsoft.com/v1.0/users/{{user_email_address}}/memberOf?%24filter=mailEnabled%20eq%20true&%24count=true' \
--header 'ConsistencyLevel: eventual'
SAP Concur
Get user id from email
NOTE: You can pass the current user's email dynamically to any API using "user.email_addr"
curl --location 'https://us2.api.concursolutions.com/profile/identity/v4/users?filter=userName eq "{{user.email_addr}}" \
--header 'Accept: application/json' \
Create Expense Report
NOTE: Notice the policy ID is hardcoded. This is intended in order to work with the current policy in the Concur Sandbox instance
curl --location 'https://us2.api.concursolutions.com/expensereports/v4/users/{{concur_user_id}}/context/TRAVELER/reports' \
--header 'Accept: application/json' \
--header 'Content-Type: application/json' \
--data '{
"policyId": "A6D42A825114472FAF402180E20B3751",
"businessPurpose":"{{business_purpose}}",
"comment": "{{comment}}",
"name": "{{report_name}}"
}'
Get Expense Reports for a User
curl --location 'https://us2.api.concursolutions.com/api/v3.0/expense/reports?user={{user.email_addr}}' \
--header 'Accept: application/json' \
Create an Expense Item in an Expense Report
NOTE: Expense Code Types can be LODNG, BUSML, TRAIN, ONLIN, MISCL - representing Lodging, Meals, Train, Internet or Miscellaneous respectively. Transaction Date must be in the format of YYYY-MM-DD. PaymentTypeID can remain hardcoded as the value below since that is the only payment method in the SAP Concur Sandbox
curl --location 'https://us2.api.concursolutions.com/api/v3.0/expense/entries?user={{user.email_addr}}' \
--header 'Accept: application/json' \
--header 'Content-Type: application/json' \
--data '{
"ExpenseTypeCode": "{{code}}",
"PaymentTypeID": "gWqVl5luZIS$p2GDptAHMavgx7CHH9",
"ReportID": "{{report_id}}",
"TransactionDate": "{{date}}",
"TransactionAmount": "{{amount}}",
"TransactionCurrencyCode": "USD"
}'
Advanced concepts
Error handling
Error handling documentation can be found here: Error Handling
Try-catch
If you are familiar with try-catch statements, you can utilize the below skeleton
- try_catch:
catch:
on_status_code:
- 400
steps:
- raise:
message: Error message to display
output_key: data_error_key
try:
- action:
output_key: group_result
action_id: action_id
progress_updates:
on_complete: On complete message
on_pending: On pending message
input_args:
arg_1: argument
Updated 2 months ago